Emulating mobile devices
Puppeteer and Playwright control headless desktop browsers that can also emulate mobile devices. And while device emulation can’t replace testing on mobile devices entirely, it’s a practical and quick-to-setup approach to testing mobile scenarios.
Device emulation is well suited to test if your site behaves correctly across multiple viewport sizes and correctly handles user-agent strings. But if your site relies on device-specific browser features, an iPhone emulation running in a Chromium browser might lead to false positives.
This guide explains how to define viewport sizes, device pixel ratio and user-agent strings using Playwright and Puppeteer.
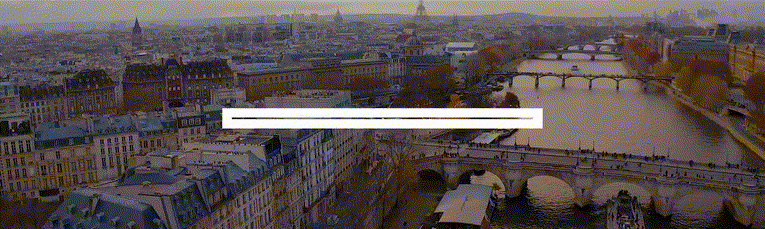
Defining the user agent string
If your site parses the user agent string to serve a different experience to mobile users, define the userAgent in your automation scripts.
Defining viewport size and pixel density
If your site follows responsive web design practices and renders elements depending on device viewport size, define a mobile viewport and pixel density.
Use built-in device registries
Playwright and Puppeteer include a built-in device registry to access mobile device characteristics quickly.
- Playwright devices
- Puppeteer devices
Leverage the pre-defined devices to emulate mobile devices.
Further reading
- Measuring page performance
- Playwright’s emulation documentation
Last updated on March 15, 2024. You can contribute to this documentation by editing this page on Github
What is Playwright?
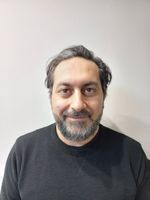
Why use Playwright?
We all know that technology moves fast, but even by modern standards, the rapid rise of Playwright is impressive.
Microsoft released Playwright in 2020 as an open-source Node library to automate Chromium, Firefox, and WebKit with a single API. Today, Playwright is one of the most popular frameworks for web automation, testing, and scraping. It provides automated control of a web browser with a few lines of code, making it particularly useful for data extraction, end-to-end testing, automating web page interaction, taking screenshots of web pages, and running automated tests for JavaScript libraries.
While similar to Puppeteer , Cypress , and Selenium , there are some differences. Let’s find out what they are.
Is Playwright a headless browser?
Not exactly. Playwright can be run in headful or headless mode (without a graphical user interface). By default, Playwright runs in headless mode, which means you won’t see what is happening in the browser when you run your script, but it will run faster. When you write and debug your scripts, it’s advisable to disable headless mode so you can see what your script is doing:
On the other hand, if performance is the most important thing for you, headless mode is the way to go since headless browsers are quicker than real browsers.
What about Puppeteer and Selenium?
Speak of headless browsers, and the names Puppeteer and Selenium immediately spring to mind. So, how do these compare to their younger sibling? Puppeteer supports only JavaScript and TypeScript and works with Chromium, with experimental support for Firefox. Playwright supports Chromium, Firefox, and Safari with WebKit. You can use many programming languages with Playwright and one extra language with Selenium (Ruby). But Playwright’s greatest advantage over Selenium is its auto-waiting function.
What languages does Playwright support?
Playwright works with some of the most popular programming languages, including JavaScript, Python, Java, and C#. Its support of Chromium, Firefox, and WebKit provides a wide range of cross-browser automation and web testing capabilities.
What platform does Playwright support?
Playwright is a cross-platform framework. The browser binaries for Chromium, Firefox, and WebKit work across three platforms: Windows (and WSL), macOS (10.14 or above), and Linux (though you may need to install additional dependencies, depending on your Linux distribution).
How do I get started with Playwright?
One thing that isn’t said enough about Playwright: its documentation is superb. There you will find out how to install Playwright to get started.
You can install the VS Code extension . After installation, open the command panel and type Install Playwright . Alternatively, you can use the command line interface (CLI) and install Playwright using the appropriate package manager for your language. For example, NPM with Node.js:
That will give you the browsers and files you need to begin:
The tests folder contains a basic example test to get you started and the tests-examples folder contains a more detailed example, with tests written to test a todo app.
Alternatively, you can simply add Playwright to your existing project by calling:
Why use Playwright for web automation and testing?
1. faster communication with the chrome devtools protocol.
Most automation solutions use the WebDriver protocol to communicate with Chromium browsers, but Playwright provides much faster and more straightforward communication with the Chrome DevTools protocol . But Playwright isn’t just for Chrome and Edge and Playwright can be configured to test sites in Firefox and Safari, as well.
2. The auto-waiting function
Cross-browser and cross-language support aside, the auto-waiting function is Playwright’s greatest advantage over Puppeteer and Selenium. You don’t have to figure out when something is clickable because Playwright performs that action for you. You can emulate mouse clicks by using await page.click() , and wait for actions in the browser to finish by using convenient APIs like await page.waitForSelector() or await page.waitForFunction() .
This unique automatic waiting feature eliminates the need to write custom waits or sleep statements in your test scripts. That means you can focus on writing high-quality tests instead of worrying about writing the perfect waiting logic.
3. Record scripts with Codegen
The Playwright documentation includes a test generator that shows you how to record your scripts with Codegen. You just need a single CLI command to kick off:
This will open up an interactive browser and the Playwright inspector. Every action in the browser will be recorded in the inspector. You can then replay and adjust the generated script. In other words, Playwright generates test script code based on your interaction with the page. That means you can author tests out of the box without having to write the script manually.
4. Great debugging capabilities
Playwright has some excellent debugging features. You can debug scripts while you run them, which is handy during local development, and you can also analyze and debug failed tests. You can open Playwright Inspector to enable debug mode with npx playwright test -- debug to debug all tests or npx playwright test example -- debug to debug one test. Alternatively, you can set the PWDEBUG environment variable to run your scripts in debug mode.
5. Native mobile emulation
Playwright supports native mobile emulation, which means you can test your web applications on mobile devices without having to set up an actual device. Playwright can emulate Safari on iOS as well as Android devices. Playwright's test runner provides numerous predefined configurations, making it easy to test your web application on multiple devices and screen sizes to ensure that it works as expected for all users without having to manually set up each configuration.
6. Comprehensive reports
Playwright provides comprehensive reporting options for test results. You can:
a) Export results as a machine-readable JSON file.
This is useful if you want to integrate Playwright tests into a larger test suite or if you want to programmatically analyze the results.
b) Export the results as a stylish HTML page.
This is a great option if you want to share the test results with other members of your team or with stakeholders. The HTML report includes detailed information about the test runs, including the number of passed and failed tests, the duration of each test, and any errors that occurred during the test run.
Why use Playwright for web scraping?
We’ve touched upon the brilliance of Playwright when it comes to web testing and automation, but its capabilities can also come in very handy when it comes to web scraping and data mining. Here’s why:
It can be very difficult to scrape some websites with regular HTML tools. Dynamic pages and browser fingerprinting are two of the biggest challenges. Playwright’s headless mode helps overcome these problems.
1. Loading dynamic pages
When it comes to pages loaded dynamically with AJAX or data rendered using JavaScript, you’ll need to render the page like a real user. HTML scrapers can’t do that. Headless browsers can. So, in such cases, you’ll need web scraping tools like Playwright Scraper or Puppeteer Scraper to load the page, execute its JavaScript, and scrape the required data.
2. Combatting browser fingerprinting
Some websites now use fingerprinting to track users and block scraping bots. A scraper that uses a headless browser can emulate the fingerprint of a real device. Without a headless browser, it’s nearly impossible to pass the various anti-bot challenges that block your access to a website. This makes using Puppeteer or Playwright Scraper your best bet when getting blocked.
Also, you can go even further and develop your own web scraper with Crawlee , a Node.js library that helps you pass those challenges automatically using Puppeteer or Playwright.
Crawlee helps you build reliable scrapers fast. Quickly scrape data, store it, and avoid getting blocked with headless browsers, smart proxy rotation, and auto-generated human-like headers and fingerprints.
Web scraping with Playwright
If you want to find out more about Playwright and web scraping, this tutorial shows you how to build a scraper with Playwright in Node.js to extract data about GitHub topics.
Related articles
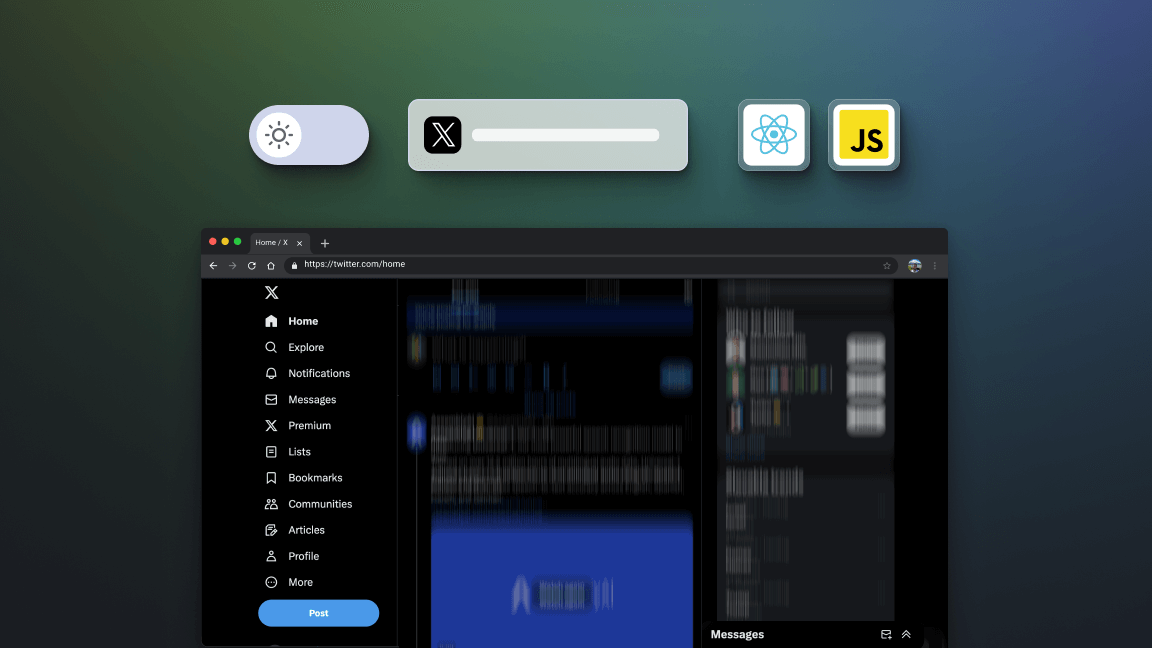
What is a dynamic web page?
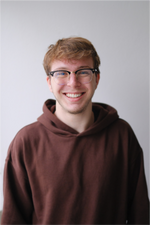
Python Playwright: a complete guide
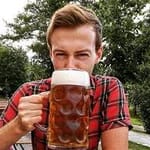
Playwright web scraping: how to scrape the web with Playwright in 2024
Get started now
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[BUG]: All webkit and Mobile Safari tests are failing with: browserContext.newPage: Protocol error #20481
NashJames commented Jan 29, 2023
mxschmitt commented Jan 30, 2023
Sorry, something went wrong.
NashJames commented Jan 31, 2023
- 👍 1 reaction
No branches or pull requests

Turn Your Curiosity Into Discovery
Latest facts.
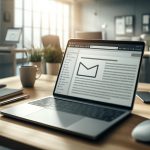
The Art of Email Marketing How to Craft Compelling Emails
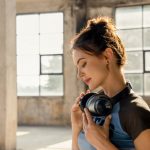
Elevate Your Audio Experience with the CuttingEdge OneOdio A10 Headphones
40 facts about elektrostal.
Written by Lanette Mayes
Modified & Updated: 02 Mar 2024

Reviewed by Jessica Corbett
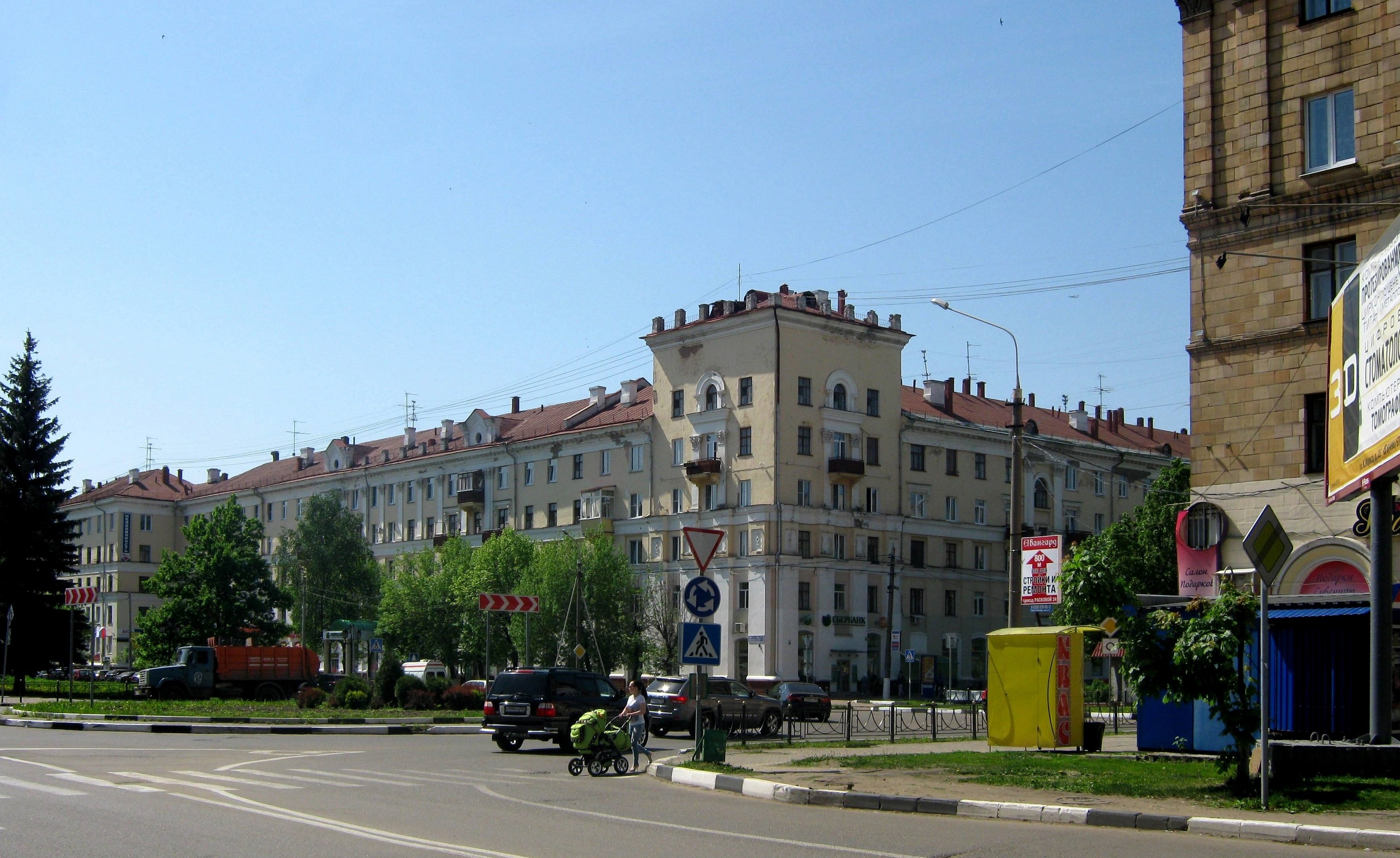
Elektrostal is a vibrant city located in the Moscow Oblast region of Russia. With a rich history, stunning architecture, and a thriving community, Elektrostal is a city that has much to offer. Whether you are a history buff, nature enthusiast, or simply curious about different cultures, Elektrostal is sure to captivate you.
This article will provide you with 40 fascinating facts about Elektrostal, giving you a better understanding of why this city is worth exploring. From its origins as an industrial hub to its modern-day charm, we will delve into the various aspects that make Elektrostal a unique and must-visit destination.
So, join us as we uncover the hidden treasures of Elektrostal and discover what makes this city a true gem in the heart of Russia.
Key Takeaways:
- Elektrostal, known as the “Motor City of Russia,” is a vibrant and growing city with a rich industrial history, offering diverse cultural experiences and a strong commitment to environmental sustainability.
- With its convenient location near Moscow, Elektrostal provides a picturesque landscape, vibrant nightlife, and a range of recreational activities, making it an ideal destination for residents and visitors alike.
Known as the “Motor City of Russia.”
Elektrostal, a city located in the Moscow Oblast region of Russia, earned the nickname “Motor City” due to its significant involvement in the automotive industry.
Home to the Elektrostal Metallurgical Plant.
Elektrostal is renowned for its metallurgical plant, which has been producing high-quality steel and alloys since its establishment in 1916.
Boasts a rich industrial heritage.
Elektrostal has a long history of industrial development, contributing to the growth and progress of the region.
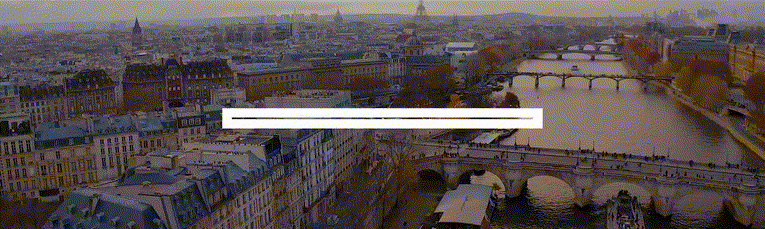
Founded in 1916.
The city of Elektrostal was founded in 1916 as a result of the construction of the Elektrostal Metallurgical Plant.
Located approximately 50 kilometers east of Moscow.
Elektrostal is situated in close proximity to the Russian capital, making it easily accessible for both residents and visitors.
Known for its vibrant cultural scene.
Elektrostal is home to several cultural institutions, including museums, theaters, and art galleries that showcase the city’s rich artistic heritage.
A popular destination for nature lovers.
Surrounded by picturesque landscapes and forests, Elektrostal offers ample opportunities for outdoor activities such as hiking, camping, and birdwatching.
Hosts the annual Elektrostal City Day celebrations.
Every year, Elektrostal organizes festive events and activities to celebrate its founding, bringing together residents and visitors in a spirit of unity and joy.
Has a population of approximately 160,000 people.
Elektrostal is home to a diverse and vibrant community of around 160,000 residents, contributing to its dynamic atmosphere.
Boasts excellent education facilities.
The city is known for its well-established educational institutions, providing quality education to students of all ages.
A center for scientific research and innovation.
Elektrostal serves as an important hub for scientific research, particularly in the fields of metallurgy, materials science, and engineering.
Surrounded by picturesque lakes.
The city is blessed with numerous beautiful lakes, offering scenic views and recreational opportunities for locals and visitors alike.
Well-connected transportation system.
Elektrostal benefits from an efficient transportation network, including highways, railways, and public transportation options, ensuring convenient travel within and beyond the city.
Famous for its traditional Russian cuisine.
Food enthusiasts can indulge in authentic Russian dishes at numerous restaurants and cafes scattered throughout Elektrostal.
Home to notable architectural landmarks.
Elektrostal boasts impressive architecture, including the Church of the Transfiguration of the Lord and the Elektrostal Palace of Culture.
Offers a wide range of recreational facilities.
Residents and visitors can enjoy various recreational activities, such as sports complexes, swimming pools, and fitness centers, enhancing the overall quality of life.
Provides a high standard of healthcare.
Elektrostal is equipped with modern medical facilities, ensuring residents have access to quality healthcare services.
Home to the Elektrostal History Museum.
The Elektrostal History Museum showcases the city’s fascinating past through exhibitions and displays.
A hub for sports enthusiasts.
Elektrostal is passionate about sports, with numerous stadiums, arenas, and sports clubs offering opportunities for athletes and spectators.
Celebrates diverse cultural festivals.
Throughout the year, Elektrostal hosts a variety of cultural festivals, celebrating different ethnicities, traditions, and art forms.
Electric power played a significant role in its early development.
Elektrostal owes its name and initial growth to the establishment of electric power stations and the utilization of electricity in the industrial sector.
Boasts a thriving economy.
The city’s strong industrial base, coupled with its strategic location near Moscow, has contributed to Elektrostal’s prosperous economic status.
Houses the Elektrostal Drama Theater.
The Elektrostal Drama Theater is a cultural centerpiece, attracting theater enthusiasts from far and wide.
Popular destination for winter sports.
Elektrostal’s proximity to ski resorts and winter sport facilities makes it a favorite destination for skiing, snowboarding, and other winter activities.
Promotes environmental sustainability.
Elektrostal prioritizes environmental protection and sustainability, implementing initiatives to reduce pollution and preserve natural resources.
Home to renowned educational institutions.
Elektrostal is known for its prestigious schools and universities, offering a wide range of academic programs to students.
Committed to cultural preservation.
The city values its cultural heritage and takes active steps to preserve and promote traditional customs, crafts, and arts.
Hosts an annual International Film Festival.
The Elektrostal International Film Festival attracts filmmakers and cinema enthusiasts from around the world, showcasing a diverse range of films.
Encourages entrepreneurship and innovation.
Elektrostal supports aspiring entrepreneurs and fosters a culture of innovation, providing opportunities for startups and business development.
Offers a range of housing options.
Elektrostal provides diverse housing options, including apartments, houses, and residential complexes, catering to different lifestyles and budgets.
Home to notable sports teams.
Elektrostal is proud of its sports legacy, with several successful sports teams competing at regional and national levels.
Boasts a vibrant nightlife scene.
Residents and visitors can enjoy a lively nightlife in Elektrostal, with numerous bars, clubs, and entertainment venues.
Promotes cultural exchange and international relations.
Elektrostal actively engages in international partnerships, cultural exchanges, and diplomatic collaborations to foster global connections.
Surrounded by beautiful nature reserves.
Nearby nature reserves, such as the Barybino Forest and Luchinskoye Lake, offer opportunities for nature enthusiasts to explore and appreciate the region’s biodiversity.
Commemorates historical events.
The city pays tribute to significant historical events through memorials, monuments, and exhibitions, ensuring the preservation of collective memory.
Promotes sports and youth development.
Elektrostal invests in sports infrastructure and programs to encourage youth participation, health, and physical fitness.
Hosts annual cultural and artistic festivals.
Throughout the year, Elektrostal celebrates its cultural diversity through festivals dedicated to music, dance, art, and theater.
Provides a picturesque landscape for photography enthusiasts.
The city’s scenic beauty, architectural landmarks, and natural surroundings make it a paradise for photographers.
Connects to Moscow via a direct train line.
The convenient train connection between Elektrostal and Moscow makes commuting between the two cities effortless.
A city with a bright future.
Elektrostal continues to grow and develop, aiming to become a model city in terms of infrastructure, sustainability, and quality of life for its residents.
In conclusion, Elektrostal is a fascinating city with a rich history and a vibrant present. From its origins as a center of steel production to its modern-day status as a hub for education and industry, Elektrostal has plenty to offer both residents and visitors. With its beautiful parks, cultural attractions, and proximity to Moscow, there is no shortage of things to see and do in this dynamic city. Whether you’re interested in exploring its historical landmarks, enjoying outdoor activities, or immersing yourself in the local culture, Elektrostal has something for everyone. So, next time you find yourself in the Moscow region, don’t miss the opportunity to discover the hidden gems of Elektrostal.
Q: What is the population of Elektrostal?
A: As of the latest data, the population of Elektrostal is approximately XXXX.
Q: How far is Elektrostal from Moscow?
A: Elektrostal is located approximately XX kilometers away from Moscow.
Q: Are there any famous landmarks in Elektrostal?
A: Yes, Elektrostal is home to several notable landmarks, including XXXX and XXXX.
Q: What industries are prominent in Elektrostal?
A: Elektrostal is known for its steel production industry and is also a center for engineering and manufacturing.
Q: Are there any universities or educational institutions in Elektrostal?
A: Yes, Elektrostal is home to XXXX University and several other educational institutions.
Q: What are some popular outdoor activities in Elektrostal?
A: Elektrostal offers several outdoor activities, such as hiking, cycling, and picnicking in its beautiful parks.
Q: Is Elektrostal well-connected in terms of transportation?
A: Yes, Elektrostal has good transportation links, including trains and buses, making it easily accessible from nearby cities.
Q: Are there any annual events or festivals in Elektrostal?
A: Yes, Elektrostal hosts various events and festivals throughout the year, including XXXX and XXXX.
Was this page helpful?
Our commitment to delivering trustworthy and engaging content is at the heart of what we do. Each fact on our site is contributed by real users like you, bringing a wealth of diverse insights and information. To ensure the highest standards of accuracy and reliability, our dedicated editors meticulously review each submission. This process guarantees that the facts we share are not only fascinating but also credible. Trust in our commitment to quality and authenticity as you explore and learn with us.
Share this Fact:
Playwright has experimental support for Android automation. This includes Chrome for Android and Android WebView.
Requirements
- Android device or AVD Emulator.
- ADB daemon running and authenticated with your device. Typically running adb devices is all you need to do.
- Chrome 87 or newer installed on the device
- "Enable command line on non-rooted devices" enabled in chrome://flags .
Known limitations
- Raw USB operation is not yet supported, so you need ADB.
- Device needs to be awake to produce screenshots. Enabling "Stay awake" developer mode will help.
- We didn't run all the tests against the device, so not everything works.
An example of the Android automation script would be:
This methods attaches Playwright to an existing Android device. Use android.launchServer() to launch a new Android server instance.
wsEndpoint string #
A browser websocket endpoint to connect to.
options Object (optional)
headers Object < string , string > (optional) #
Additional HTTP headers to be sent with web socket connect request. Optional.
slowMo number (optional) #
Slows down Playwright operations by the specified amount of milliseconds. Useful so that you can see what is going on. Defaults to 0 .
timeout number (optional) #
Maximum time in milliseconds to wait for the connection to be established. Defaults to 30000 (30 seconds). Pass 0 to disable timeout.
- Promise < AndroidDevice > #
Returns the list of detected Android devices.
host string (optional) Added in: v1.22 #
Optional host to establish ADB server connection. Default to 127.0.0.1 .
omitDriverInstall boolean (optional) Added in: v1.21 #
Prevents automatic playwright driver installation on attach. Assumes that the drivers have been installed already.
port number (optional) Added in: v1.20 #
Optional port to establish ADB server connection. Default to 5037 .
- Promise < Array < AndroidDevice >> #
launchServer
Launches Playwright Android server that clients can connect to. See the following example:
Server Side:
Client Side:
adbHost string (optional) #
adbPort number (optional) #
deviceSerialNumber string (optional) #
Optional device serial number to launch the browser on. If not specified, it will throw if multiple devices are connected.
omitDriverInstall boolean (optional) #
port number (optional) #
Port to use for the web socket. Defaults to 0 that picks any available port.
wsPath string (optional) #
Path at which to serve the Android Server. For security, this defaults to an unguessable string.
Any process or web page (including those running in Playwright) with knowledge of the wsPath can take control of the OS user. For this reason, you should use an unguessable token when using this option.
- Promise < BrowserServer > #
setDefaultTimeout
This setting will change the default maximum time for all the methods accepting timeout option.
timeout number #
Maximum time in milliseconds
- launchServer
- setDefaultTimeout
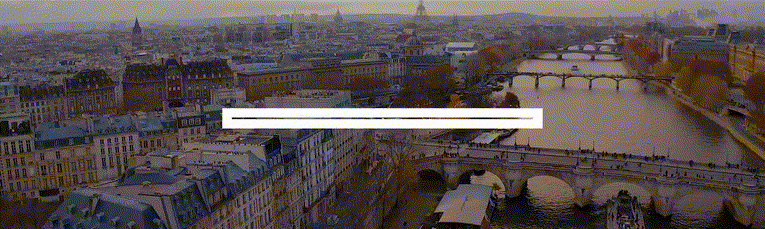
IMAGES
VIDEO
COMMENTS
Playwright's WebKit version matches the recent WebKit trunk build, before it is used in Apple Safari and other WebKit-based browsers. This gives a lot of lead time to react on the potential browser update issues. Playwright doesn't work with the branded version of Safari since it relies on patches. Instead you can test against the recent WebKit ...
Since Playwright is trying to be more testing-friendly, it would be really great to have real mobile device support. More and more users are coming to our websites from mobile devices, it is nearly 50:50 for us. Most of our bugs on mobile devices are not discoverable via simple mobile emulation.
Test Mobile Web. Native mobile emulation of Google Chrome for Android and Mobile Safari. The same rendering engine works on your Desktop and in the Cloud. Resilient • No flaky tests. Auto-wait. Playwright waits for elements to be actionable prior to performing actions. It also has a rich set of introspection events.
Playwright can also run on emulated tablet and mobile devices. See the registry of device parameters for a complete list of selected desktop, tablet and mobile devices. import {defineConfig, devices } from '@playwright/test'; ... [Mobile Safari] › example.spec.ts:3:1 › basic test (2s) [Microsoft Edge] ...
Puppeteer and Playwright control headless desktop browsers that can also emulate mobile devices. And while device emulation can't replace testing on mobile devices entirely, it's a practical and quick-to-setup approach to testing mobile scenarios. Device emulation is well suited to test if your site behaves correctly across multiple viewport sizes and correctly handles user-agent strings.
This code snippet navigates to playwright.dev in Chromium and WebKit, and saves 2 screenshots. Mobile and geolocation emulation. PDF generation with Chromium. Video recording. JavaScript evaluation in browser context. Logging network requests. Modifying network requests. End-to-End test a todo application.
Playwright is a web test automation library that tests against the underlying engine for the foremost popular browsers: Chromium for Chrome and Edge, Webkit for Safari, and Gecko for Firefox. Test scripts are often written in JavaScript, Python, C#, and Go. Playwright leverages the DevTools protocol.
Playwright is a framework for Web Testing and Automation. It allows testing Chromium, Firefox and WebKit with a single API. - GitHub - microsoft/playwright: Playwright is a framework for Web Testing and Automation. ... This snippet emulates Mobile Safari on a device at given geolocation, navigates to maps.google.com, performs the action and ...
The steps to run a Playwright test using the device mode on Automate are same as the ones you perform for integration. The only difference is that you have to specify Playwright emulation configurations under playwrightConfigOptions in the browserstack.yml file. For example, you may only want to specify that the test runs on iPhone 6 and prefer ...
The site being tested is responsive so things like images might be visible on desktop but not on mobile so I need a way to put a condition in my test to assert it's visible if desktop but not if mobile.
Playwright supports native mobile emulation, which means you can test your web applications on mobile devices without having to set up an actual device. Playwright can emulate Safari on iOS as well as Android devices. Playwright's test runner provides numerous predefined configurations, making it easy to test your web application on multiple ...
Playwright Test was created specifically to accommodate the needs of end-to-end testing. Playwright supports all modern rendering engines including Chromium, WebKit, and Firefox. Test on Windows, Linux, and macOS, locally or on CI, headless or headed with native mobile emulation of Google Chrome for Android and Mobile Safari. You will learn
Guessing the duplicate webkit caused the issue. The only thing I can think created the mismatched browsers was using pnpm dlx playwright install --with-deps firefox chromium webkit chrome msedge when v1.30. was available and I had v1.28. of @playwright/test. I only then learned pnpm exec was reccommended here after receiving the mismatched versions warnings then.
Playwright is a framework for automating web browser interactions with JavaScript. It supports multiple browsers, platforms and devices, and offers a rich API for testing, debugging and monitoring web apps. Learn more at playright.dev.
Cut wait times with shorter builds that give you test results in minutes. With parallel testing, you can speed up the release process while expanding test and browser coverage. Verify end-to-end functionality on every commit, and catch bugs early in the cycle. Optimizely runs 15,000 tests every 45 minutes on Automate to deploy every 4 hours.
In 1938, it was granted town status. [citation needed]Administrative and municipal status. Within the framework of administrative divisions, it is incorporated as Elektrostal City Under Oblast Jurisdiction—an administrative unit with the status equal to that of the districts. As a municipal division, Elektrostal City Under Oblast Jurisdiction is incorporated as Elektrostal Urban Okrug.
40 Facts About Elektrostal. Elektrostal is a vibrant city located in the Moscow Oblast region of Russia. With a rich history, stunning architecture, and a thriving community, Elektrostal is a city that has much to offer. Whether you are a history buff, nature enthusiast, or simply curious about different cultures, Elektrostal is sure to ...
Prevents automatic playwright driver installation on attach. Assumes that the drivers have been installed already. port number (optional)# Port to use for the web socket. Defaults to 0 that picks any available port. wsPath string (optional)# Path at which to serve the Android Server. For security, this defaults to an unguessable string.
Industry: Remediation and Other Waste Management Services , Metal Ore Mining , Residential Building Construction , Iron and Steel Mills and Ferroalloy Manufacturing , Recycling, waste materials See All Industries, Iron ores, Metal ores, nec, Operative builders, Blast furnaces and steel mills Galvanized pipes, plates, sheets, etc.: iron and steel See Fewer Industries
Elektrostal. Elektrostal ( Russian: Электроста́ль) is a city in Moscow Oblast, Russia. It is 58 kilometers (36 mi) east of Moscow. As of 2010, 155,196 people lived there.